Table of Contents
- About the Project
- Technologies and Frameworks
- Scrum Agile Methodologies
- Soft Skills in Action
- Authentication
- Payment Integration
- Additional Libraries and Services
- Developing the Website
- Entities Overview
- Controllers Overview
- Services Overview
- Conclusion
About the project
This project was the capstone for my Software Development Associate of Applied Science degree, completed collaboratively with two other students. Our objective was to develop a comprehensive web application for a local fitness instructor, integrating various functionalities essential for managing a service-oriented eCommerce business.
Built with ASP.NET Core MVC, the application includes user registration and authentication, class and event management, newsletter functionalities, subscription services, an online store, and administrative tools for efficient business operations. This functionality required frequent, structured interactions with our client to fully understand and refine project requirements.
Scrum Agile Methodologies
To ensure the project met evolving requirements, our team adopted Scrum Agile methodologies, organizing our work into sprints and holding regular stand-ups to assess progress and address any challenges. Through consistent client meetings, we gathered feedback, discussed changes, and aligned our development priorities with the client’s goals. This iterative process enabled us to rapidly develop the application over two academic terms, ensuring functionality was delivered and adjusted efficiently in response to client needs.
Soft Skills in Action
In addition to technical development, this project required strong collaboration and communication skills. Each team member took on distinct roles to manage tasks, collaborate effectively, and adapt to changes in scope or requirements. Our success was in large part due to our ability to remain adaptable, communicate openly, and support one another’s contributions to the project.
Technologies and Frameworks
The project extensively utilizes ASP.NET Core MVC, which provides a structured approach to web application development, promoting separation of concerns and enhancing modularity. For data access, we employed Entity Framework Core (EF Core) with Pomelo.EntityFrameworkCore.MySql to interact with a MySQL database. This Object-Relational Mapping (ORM) framework facilitated seamless data manipulation and querying, enabling efficient database operations.
Through this project, we not only honed our technical abilities but also strengthened essential teamwork and project management skills—valuable competencies that will support future client-centered projects.
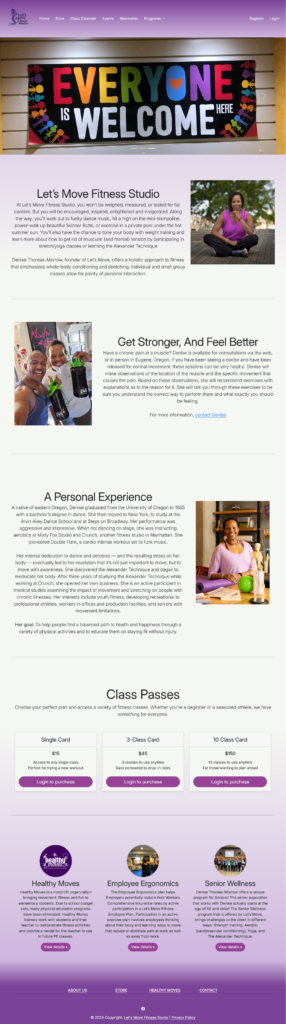
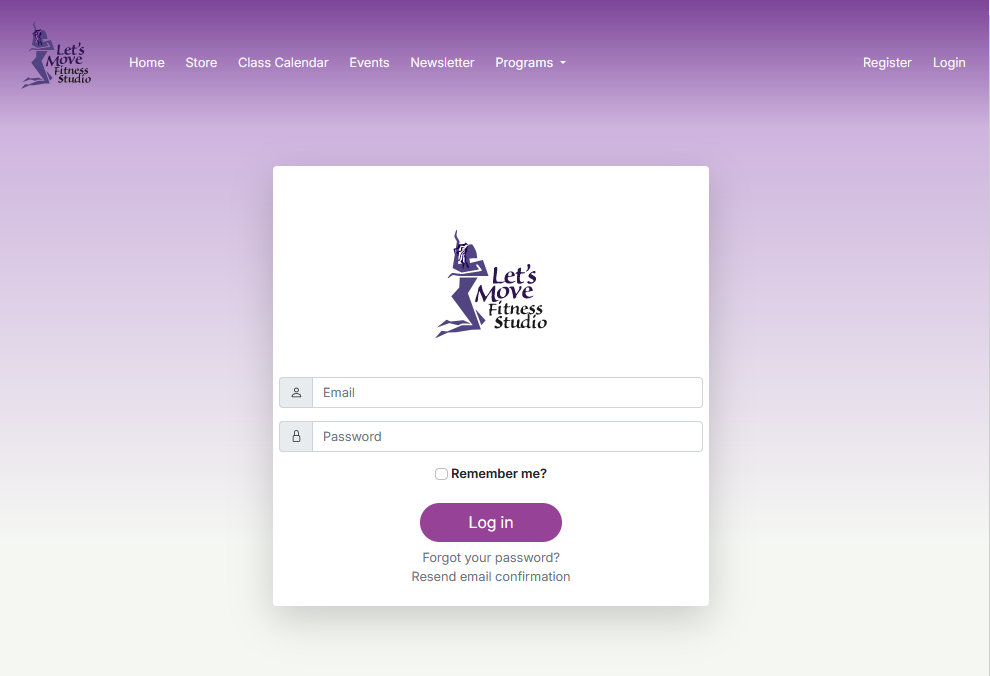
Authentication
User authentication and authorization were managed using ASP.NET Core Identity, incorporating features such as email confirmation, strong password policies, and role-based access control.
Payment Integration
To handle secure payment processing, we integrated the Braintree payment gateway, enabling seamless and reliable transactions for both subscriptions and store purchases. This eCommerce capability enhanced the user experience by providing a smooth and secure checkout process.
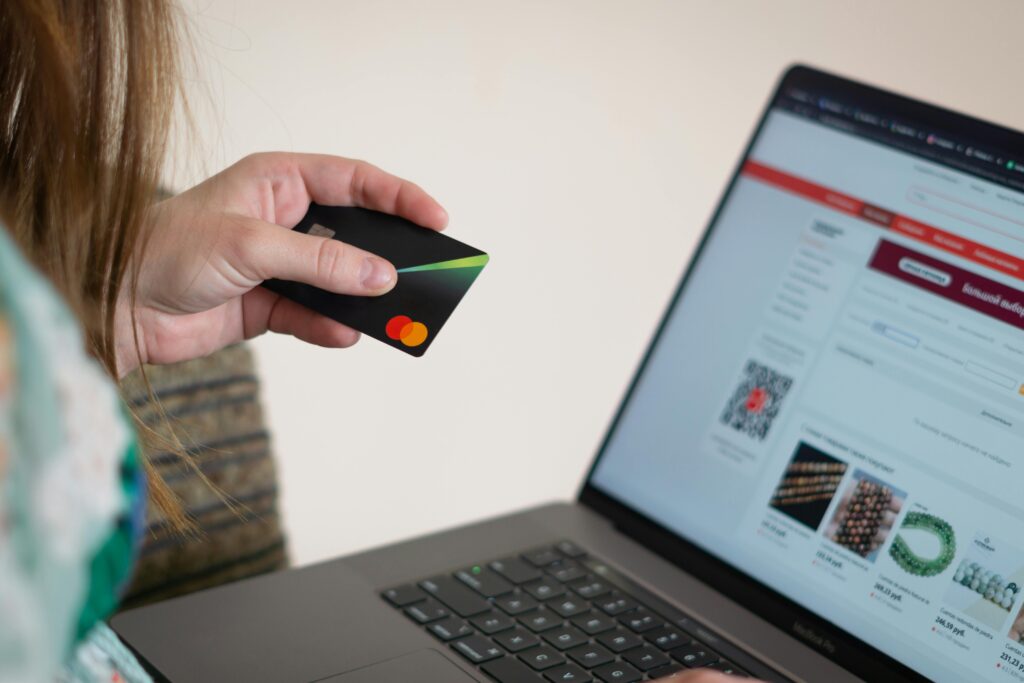
Additional Libraries and Services
Several additional libraries and services were incorporated to extend the application’s functionality:
The Humanizer library was implemented to improve the readability of time-related data, adding a more user-friendly touch to time-based information throughout the application.
CsvHelper and EPPlus were used for handling CSV files and generating Excel files, supporting data import/export features essential for business administration.
MailKit and MimeKit were used for sending email notifications, including newsletters, subscription confirmations, and other user engagements.
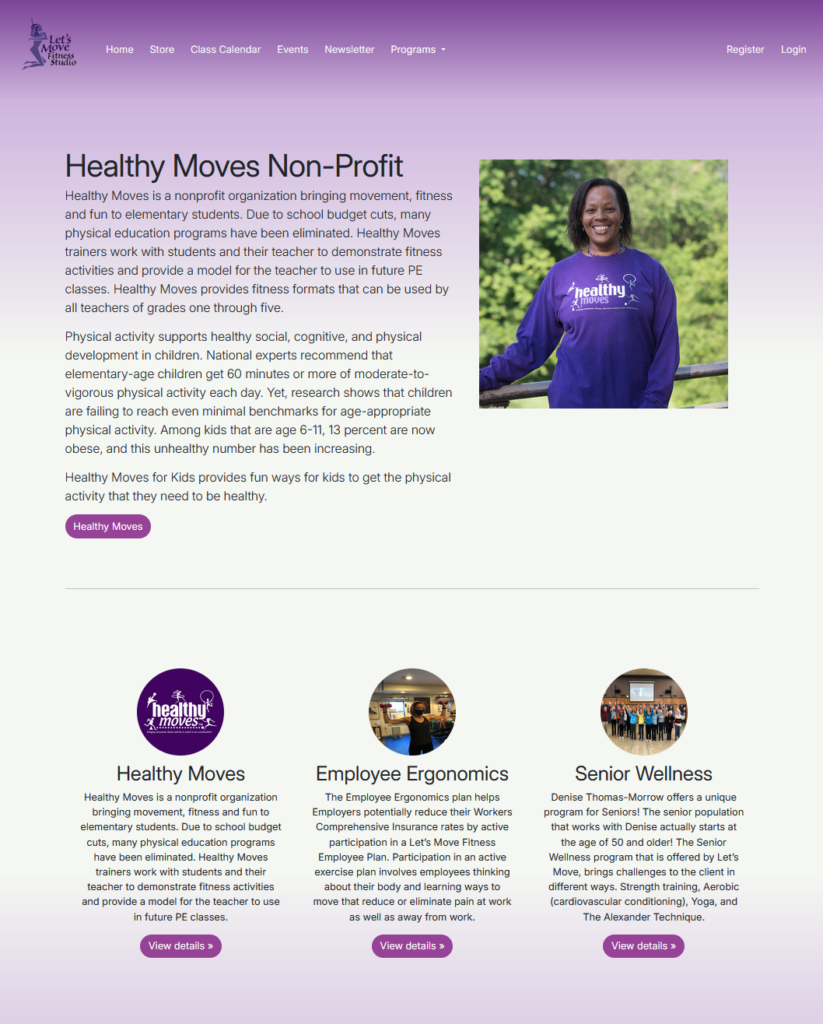
This project demonstrates our ability to integrate and manage diverse technologies and frameworks in a sophisticated, service-based eCommerce web application. By employing ASP.NET Core MVC, EF Core, and a range of essential libraries, we developed a robust, maintainable solution tailored to the needs of a fitness business. This experience not only strengthened our technical skills but also demonstrated our teamwork, our ability to manage complex requirements, and our commitment to delivering a high-quality, user-centric product.
Developing the Website
Entities Overview
The Entities in this project form the backbone of the application’s data structure. Each entity represents a core concept within the application, capturing essential data and relationships. These classes are designed with data annotations for validation, ensuring data integrity and consistency. They leverage Entity Framework Core for ORM, which simplifies database interactions and enables seamless integration with the application’s services.
Each entity is annotated with relevant data validation attributes, such as [Required]
for mandatory fields, and [NotMapped]
for properties that don’t map directly to the database. This approach enforces data integrity at the model level, allowing the system to manage complex interactions effectively.
This application includes several other entities to support additional features, such as newsletters, store management, and user profiles. What is offered below is a detailed view of key components, leaving other components out for brevity.
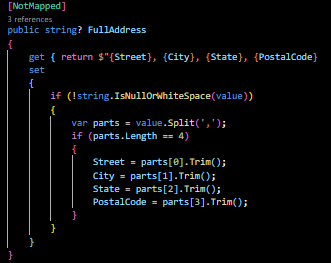
Address.cs
Key Feature: The FullAddress
property provides a computed, user-friendly format for addresses, facilitating ease of access and presentation. This property is marked with [NotMapped]
, meaning it’s not stored in the database but available for convenient use within the application.
Purpose: Stores user address information for shipping and billing purposes.
Class.cs
Key Feature: Contains relationships with Address
for location and ClassRegistration
for tracking user enrollments. The entity also includes logic for virtual sessions by conditionally assigning the AddressId
.
Purpose: Represents a class (session or event) that users can register for, either virtual or in-person.
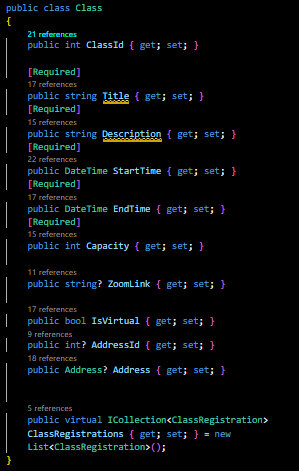
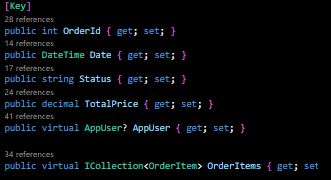
Order.cs
Purpose: Represents a user’s order, including items purchased, total price, and order status.
Key Feature: Automatically sets the Date
and Status
when a new order is created, ensuring consistency across all orders. The relationship with OrderItems
enables dynamic aggregation of order details.
OrderItem.cs
Purpose: Represents an item within an order.
Key Feature: Stores the Quantity
and Price
for each item, linking back to the associated Order
and StoreItem
. This setup provides the ability to track individual items within a complex order.
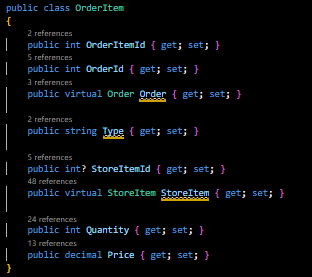
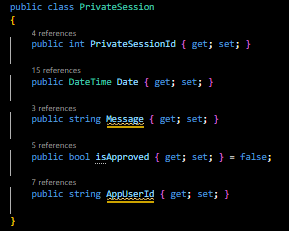
PrivateSession.cs
Key Feature: Includes an approval mechanism with the isApproved
flag, supporting asynchronous approval workflows.
Purpose: Tracks private session requests made by users.
Controllers Overview
The Controllers in this application handle core functionality, managing user interactions, data retrieval, and business logic. Below is a selection of key controllers that exemplify essential aspects of the application’s functionality. Other controllers support additional features but are omitted here for brevity.
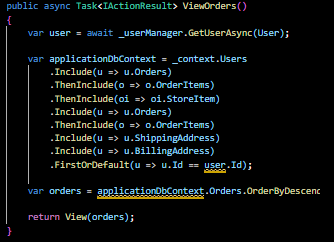
AccountController: Manages user-specific actions, such as viewing orders and registered classes. This controller demonstrates effective handling of complex data relationships and identity-based permissions, ensuring a secure and customized user experience.
CartController: Handles the shopping cart, allowing users to add, update, or remove items. This controller includes real-time pricing logic and custom handling for private sessions, demonstrating advanced cart management with asynchronous programming for smooth, efficient operations.
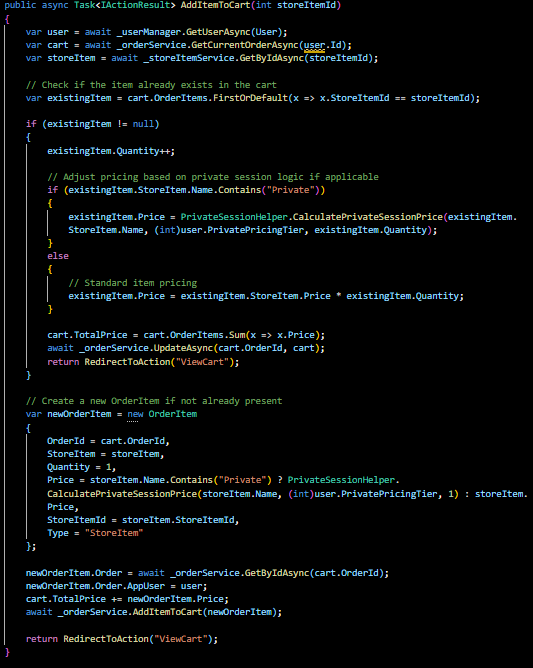
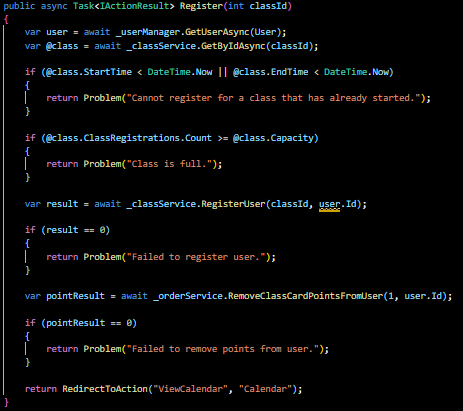
ClassController: Facilitates the registration process for classes, including validation and capacity checks. Admin functions for managing user points add a layer of role-based functionality, showcasing the application’s versatility in handling both user and admin interactions.
PaymentController: This controller handles the application’s secure payment processing through the Braintree gateway, providing a smooth, user-friendly checkout experience. The PaymentController
manages several key tasks, such as generating client tokens for frontend security, handling payment confirmations, and updating the order status based on transaction success or failure. Comprehensive error handling is implemented to ensure that any issues during payment are gracefully managed, preventing partial transactions or data inconsistencies.
To ensure reliability, the PaymentController
also includes rollback mechanisms. If a payment fails, any points added or cart items reserved are reversed, preserving both inventory and user account accuracy. Additionally, the controller includes logic for handling different types of purchases, like single sessions, bundles, and recurring subscriptions. This flexibility allows the application to support diverse business models, including one-time and subscription-based payments.
Features:
Integration with External Services: Securely connects to third party service, Braintree, and manages API interactions.
Asynchronous Programming: To maintain optimal performance, the payment process runs asynchronously, ensuring a seamless user experience without blocking other functions.
User-Centric Design: The controller’s streamlined flow prioritizes user experience, with real-time feedback on transaction statuses and intuitive redirection based on payment outcomes.
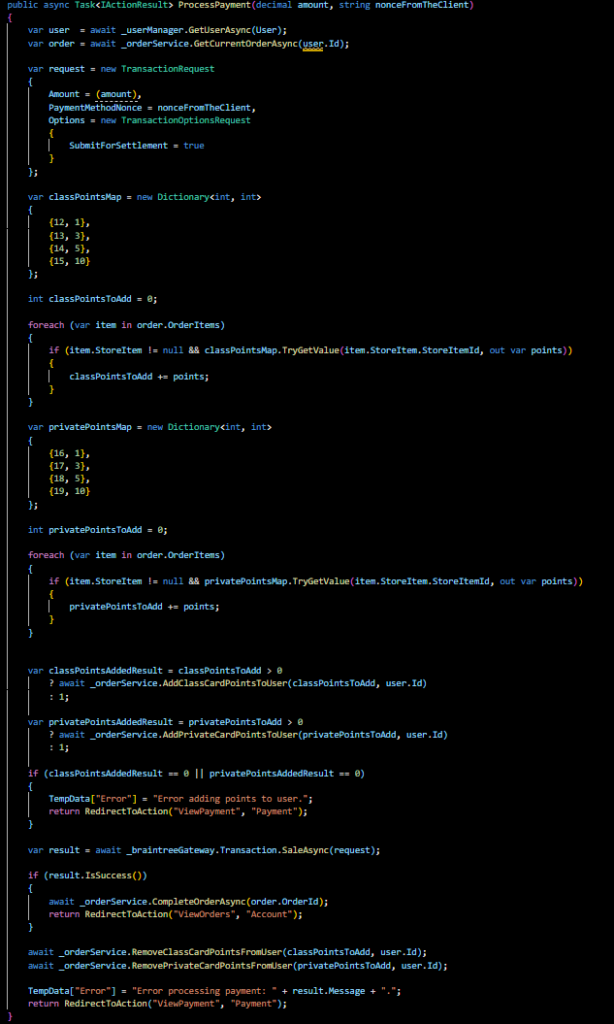
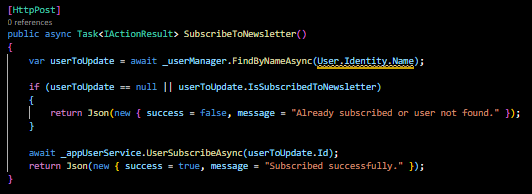
NewsletterController: Manages newsletter subscriptions. This controller demonstrates both user engagement functionality and data management capabilities, including export features for administrative ease.
Important to the NewsletterController is the SubscribersExcel method which allows administrators to export subscriber data to excel.
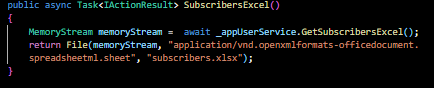
Services Overview
The Services layer encapsulates the business logic and interacts directly with the data repositories, allowing controllers to focus on handling HTTP requests. This layer enhances the application’s modularity, maintainability, and testability. Below is an overview of the core services that support the application’s most essential functionalities.
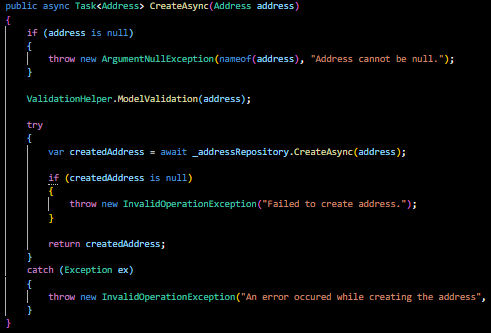
AddressService: Manages address-related operations, including CRUD functionality and validation to ensure data integrity. This service also supports dynamic filtering and sorting, providing flexibility in data retrieval and display.
AppUserService: Facilitates user management, including registration and subscription functionalities. This service also allows admins to export user data to Excel, showcasing a practical example of data handling and user engagement features.
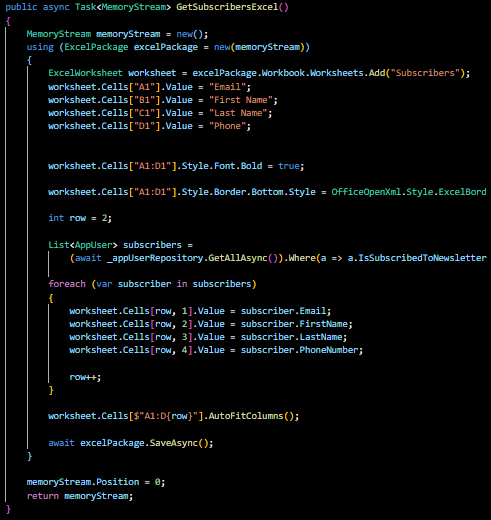
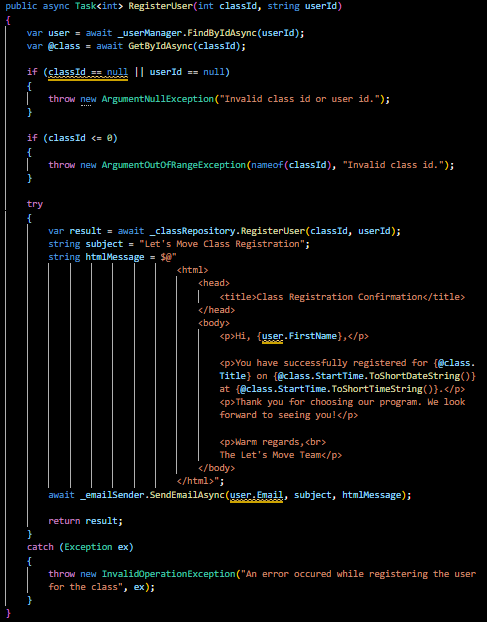
ClassService: Handles class registrations, capacity checks, and user notifications via email. This service integrates with an email provider to keep users informed of their registration status, enhancing the user experience. It also includes sorting and filtering functionalities, making it easy to retrieve relevant class information.
OrderService: Central to the e-commerce functionality, this service manages orders, cart operations, and reward points. It demonstrates advanced logic for handling transactions, including error handling, and integrates with an external payment gateway for seamless checkout processes.
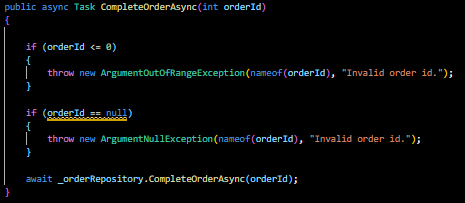
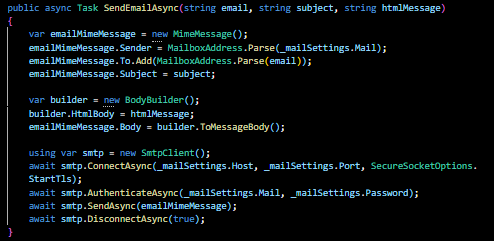
EmailSender: Manages the application’s email notifications using MailKit, including configuration for SMTP settings. This service is crucial for sending transactional emails, such as registration confirmations and newsletter subscriptions, which add a professional touch to user communications.
Conclusion
Developing this comprehensive web application presented an invaluable opportunity to refine my technical and collaborative skills in a real-world context. By building out a robust architecture with ASP.NET Core MVC and utilizing agile principles, my team and I crafted a scalable, maintainable solution that met our client’s requirements for a service-based eCommerce platform. This project honed my understanding of MVC structure, ORM with Entity Framework, and essential integrations, such as Braintree for secure payments. Working through both expected and unforeseen challenges alongside my team taught me the importance of flexibility, clear communication, and iterative development in delivering a polished, functional product. This experience has been instrumental in deepening my readiness for future full-stack development projects and in my commitment to delivering impactful, client-focused solutions.
Special thanks to my project partners for their collaboration on this project.
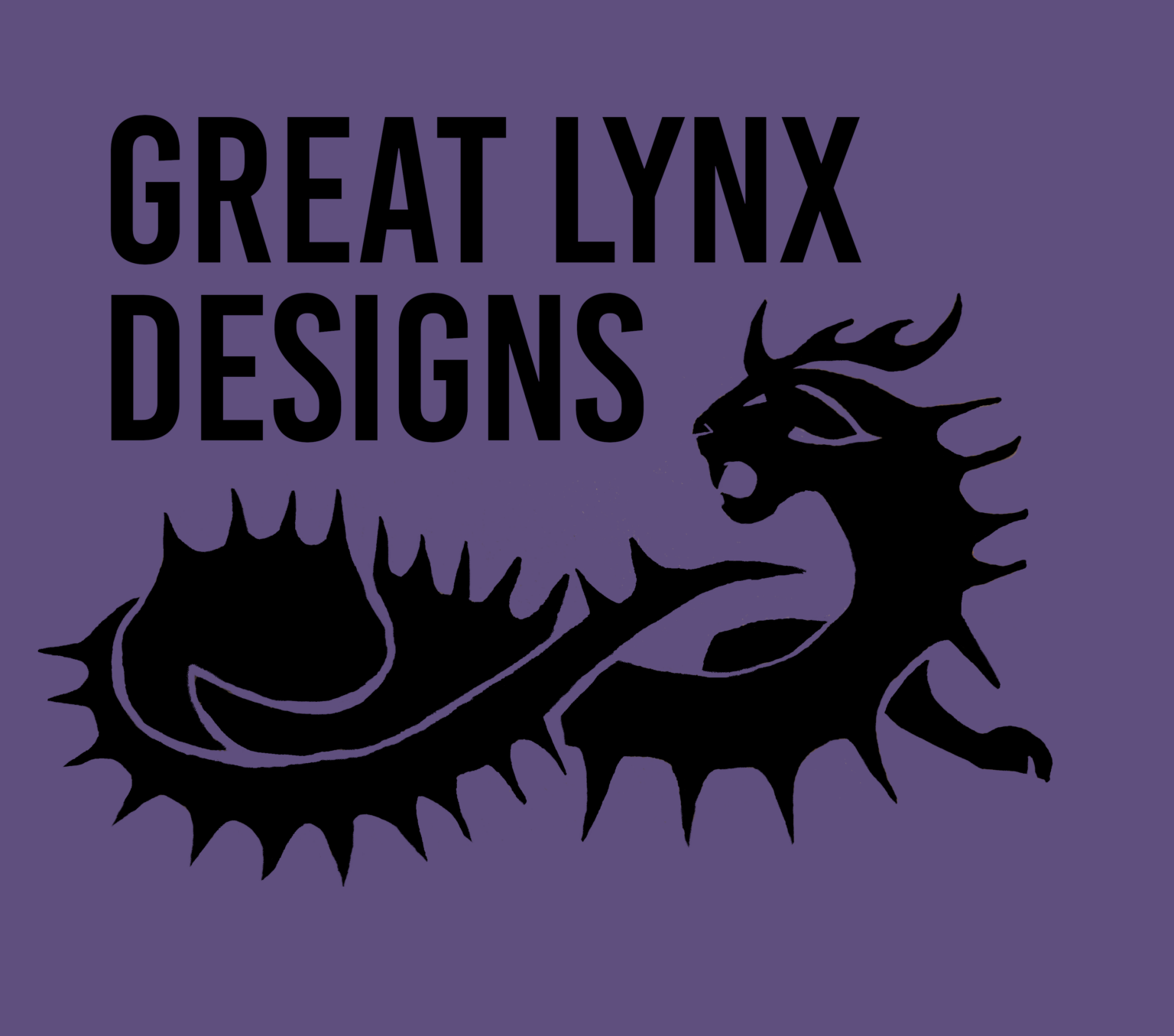
Let’s put ourselves out there
Let’s create your website, the possibilities are endless. With our collaboration, let’s go find those sales or views you’re looking for.